Payment WebElement
The Payment WebElement is a fully customizable embeddable component that lets you securely collect payment details from a secure form.
Below is an example of the default component with card
and us_bank_account
enabled.
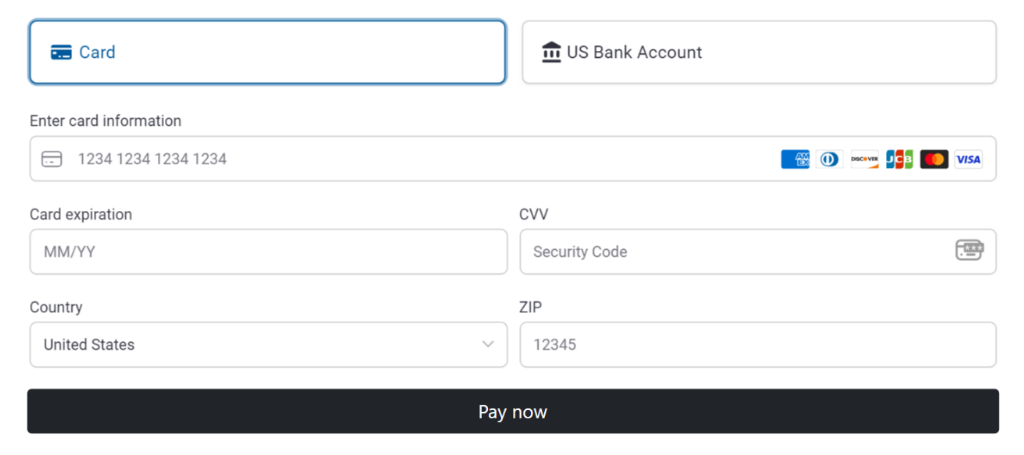
Create a Payment WebElement
Use the following method to create an instance of the Payment WebElement. The options parameter allows you to customize the Payment WebElement.
var paymentWebElement = webElementGroup.create('payment', {
layout: 'tab',
defaultValues: {
billingDetails: {
name: 'John Doe',
address: {
postalCode: '90583'
}
}
},
terms: {
card: 'auto',
usBankAccount: 'auto'
},
wallets: {
applePay: 'auto',
googlePay: 'auto'
}
});
Mount the Payment WebElement
Once the Payment WebElement is created, mount it to a DOM element in your page by specifying its ID.
paymentWebElement.mount('#payment-element');
Listening to the Events from the Payment WebElement
Our Payment Web Element triggers certain events based on actions performed by the user or internally by the SDK.
"Ready" event
This ready
event will be triggered when the Payment WebElement has been rendered an is ready to receive user inputs.
paymentWebElement.on('ready', (event) => {
// Example of a "ready" event
// {
// "avaiablePaymentMethods": {
// "paymentMethodTypes": [
// "card",
// "us_bank_account"
// ],
// "wallets": {
// "googlePay": true,
// "applePay": true
// }
// },
// "webElement": "payment"
// }
});
"Information" event
The information
event will be triggered when a user enters their information in the Payment WebElement. You'll receive multiple events as the user completes each field in the Payment WebElement form.
paymentWebElement.on('information', (event) => {
// Example of a Credit Card Information event
// {
// "webElement": "payment",
// "billingDetails": {
// "country": "US",
// "postalCode": "91351"
// },
// "type": "card",
// "card": {
// "brand": "visa",
// "last4": "1111"
// }
// }
// Example of a US Bank Account Information event
// {
// "webElement": "payment",
// "billingDetails": {
// "email": "[email protected]",
// "name": "John Doe"
// },
// "type": "us_bank_account",
// "usBankAccount": {
// "last4": "6789"
// }
// }
// Example of a Google Pay Information event (When configured with setting to require billing/shipping/phone/email)
// {
// "type": "card",
// "card": {
// "brand": "visa",
// "last4": "1111",
// "wallet": "google_pay"
// },
// "billingDetails": {
// "name": "Card Holder Name",
// "email": "[email protected]",
// "phone": "+1 650-555-5555",
// "address": {
// "city": "Mountain View",
// "country": "US",
// "line1": "1600 Amphitheatre Parkway",
// "line2": "",
// "postalCode": "94043",
// "state": "CA"
// }
// },
// "shippingDetails": {
// "email": "[email protected]",
// "address": {}
// },
// "shippingRate": {},
// "lineItems": [
// {
// "name": "Subtotal",
// "amount": "0.00"
// }
// ],
// "totalAmount": "0.00",
// "webElement": "payment"
// }
});
"Change Navigation" event
This changeNavigation
event will be triggered when a user clicks on a payment method tab on the Payment WebElement. The Event will contain a property called navigationName
. The possible values for this property are card
, us_bank_account
, apple_pay
, google_pay
paymentWebElement.on('changeNavigation', (event) => {
// Example of a "changeNavigation" event
// {
// "navigationName": "us_bank_account"
// }
});
"Cancel" event
This cancel
event will be triggered when a user cancels a payment on the Payment WebElement (e.g. when closing an apple pay or google pay payment sheet),
paymentWebElement.on('cancel', (event) => {
// Example of a "cancel" event
// {
// "webElement": "payment"
// }
});
"Shipping Address Change" event
This shippingAddressChange
event will be triggered when a customer changes the shipping address on a wallet's payment sheet (e.g. apple pay/ google pay)
paymentWebElement.on('shippingAddressChange', (event) => {
// Example of a "shippingAddressChange" event
// {
// "shippingDetails": {
// "address": {
// "city": "Montreal",
// "country": "CA",
// "postalCode": "H3B",
// "state": "Quebec"
// }
// },
// "webElement": "payment"
// }
// Use this event to update the wallet payment sheet with new shipping rates options
// after the user has selected a different address from their wallet
// let options = { ... }
// paymentElement.updatePaymentSheet(options)
});
Other Methods
The Payment WebElement has other methods that can be invoked to help you build your ideal checkout flow. Please refer to the Payment WebElement Methods for more information on the following 2 methods:
- update() - sets default values to be rendered by the Payment Web Element
- updatePaymentSheet() - updates the wallet payment sheet (e.g. line items, shipping rates)
Updated 5 months ago